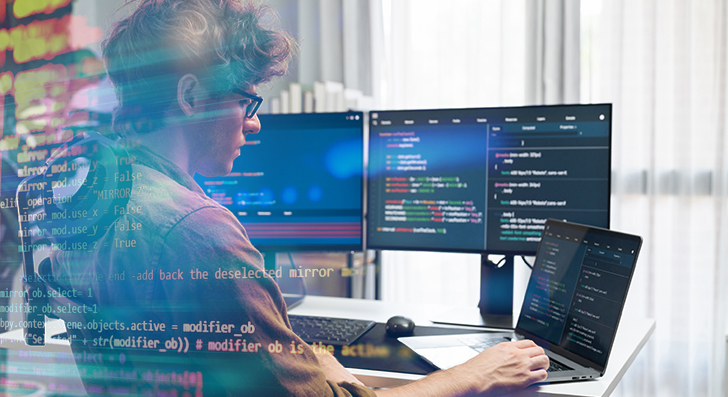
Scalability suggests your application can handle advancement—extra people, far more knowledge, and even more site visitors—with out breaking. Like a developer, developing with scalability in your mind saves time and tension afterwards. Right here’s a transparent and functional manual to assist you start by Gustavo Woltmann.
Style and design for Scalability from the Start
Scalability is just not anything you bolt on later—it ought to be component within your prepare from the beginning. Many apps fail whenever they grow rapid since the first design and style can’t manage the extra load. Being a developer, you have to Assume early about how your technique will behave stressed.
Commence by building your architecture being flexible. Keep away from monolithic codebases where by every little thing is tightly related. Instead, use modular layout or microservices. These styles break your app into lesser, independent elements. Each and every module or assistance can scale on its own without having impacting The complete system.
Also, think of your database from day one particular. Will it have to have to handle a million end users or merely 100? Pick the correct style—relational or NoSQL—based on how your information will expand. Prepare for sharding, indexing, and backups early, Even when you don’t have to have them yet.
An additional crucial place is to prevent hardcoding assumptions. Don’t compose code that only performs underneath latest ailments. Give thought to what would happen if your user foundation doubled tomorrow. Would your app crash? Would the database slow down?
Use design patterns that support scaling, like information queues or party-pushed devices. These enable your app tackle extra requests without the need of having overloaded.
After you Make with scalability in your mind, you're not just getting ready for success—you are decreasing future problems. A perfectly-prepared program is easier to maintain, adapt, and mature. It’s superior to get ready early than to rebuild later on.
Use the correct Database
Deciding on the suitable database is a vital Component of creating scalable applications. Not all databases are constructed the same, and utilizing the Improper you can sluggish you down or perhaps induce failures as your application grows.
Begin by being familiar with your facts. Could it be extremely structured, like rows inside of a table? If yes, a relational databases like PostgreSQL or MySQL is an effective in good shape. These are typically powerful with interactions, transactions, and consistency. Additionally they support scaling approaches like go through replicas, indexing, and partitioning to handle far more visitors and facts.
In case your details is much more adaptable—like person activity logs, product or service catalogs, or documents—take into account a NoSQL option like MongoDB, Cassandra, or DynamoDB. NoSQL databases are greater at managing huge volumes of unstructured or semi-structured details and may scale horizontally additional simply.
Also, consider your read through and generate patterns. Will you be performing a great deal of reads with much less writes? Use caching and read replicas. Do you think you're managing a weighty generate load? Explore databases which will handle large produce throughput, or even occasion-based mostly facts storage units like Apache Kafka (for short-term details streams).
It’s also intelligent to Consider forward. You might not have to have Sophisticated scaling functions now, but picking a databases that supports them suggests you received’t want to change later on.
Use indexing to hurry up queries. Keep away from avoidable joins. Normalize or denormalize your details depending on your access patterns. And usually keep track of database effectiveness when you improve.
To put it briefly, the ideal databases relies on your application’s framework, pace demands, And just how you assume it to mature. Choose time to select correctly—it’ll help save a great deal of issues later on.
Enhance Code and Queries
Quick code is key to scalability. As your application grows, just about every smaller hold off provides up. Badly created code or unoptimized queries can slow down performance and overload your procedure. That’s why it’s imperative that you Make successful logic from the start.
Start by crafting clean, very simple code. Prevent repeating logic and remove something unnecessary. Don’t pick the most intricate Answer if a straightforward just one operates. Keep your features small, targeted, and easy to check. Use profiling resources to uncover bottlenecks—spots in which your code takes far too extended to operate or employs an excessive amount of memory.
Subsequent, evaluate your database queries. These normally sluggish matters down over the code alone. Be sure each query only asks for the info you really will need. Prevent Choose *, which fetches anything, and rather pick out particular fields. Use indexes to hurry up lookups. And avoid undertaking a lot of joins, Particularly throughout significant tables.
Should you discover precisely the same details getting asked for many times, use caching. Retailer the final results quickly utilizing equipment like Redis or Memcached this means you don’t need to repeat high-priced functions.
Also, batch your database operations if you can. In lieu of updating a row one by one, update them in groups. This cuts down on overhead and helps make your application additional efficient.
Remember to check with massive datasets. Code and queries that get the job done great with one hundred data could crash every time they have to handle 1 million.
In brief, scalable apps are quickly apps. Maintain your code restricted, your queries lean, and use caching when essential. These methods enable your software stay smooth and responsive, even as the load increases.
Leverage Load Balancing and Caching
As your app grows, it has to handle more customers and much more site visitors. If every little thing goes by means of a single server, it's going to promptly turn into a bottleneck. That’s wherever load balancing and caching can be found in. These two resources assist keep your application speedy, secure, and scalable.
Load balancing spreads incoming website traffic across several servers. Rather than 1 server undertaking each of the function, the load balancer routes customers to different servers dependant on availability. What this means is no solitary server gets overloaded. If a person server goes down, the load balancer can send out traffic to the Other people. Tools like Nginx, HAProxy, or cloud-dependent remedies from AWS and Google Cloud make this simple to create.
Caching is about storing information quickly so it may be reused rapidly. When buyers ask for exactly the same information and facts yet again—like a product web site or possibly a profile—you don’t have to fetch it within the database each and every time. You can provide it in the cache.
There's two widespread kinds of caching:
one. Server-side caching (like Redis or Memcached) outlets info in memory for fast accessibility.
two. Consumer-facet caching (like browser caching or CDN caching) retailers static data files near to the user.
Caching lowers click here database load, enhances velocity, and tends to make your application much more successful.
Use caching for things that don’t adjust often. And constantly make sure your cache is up-to-date when details does transform.
In brief, load balancing and caching are uncomplicated but powerful equipment. Alongside one another, they help your app cope with much more end users, continue to be quick, and Recuperate from complications. If you plan to expand, you require each.
Use Cloud and Container Instruments
To make scalable applications, you may need applications that let your app expand quickly. That’s where cloud platforms and containers come in. They give you versatility, lessen set up time, and make scaling A great deal smoother.
Cloud platforms like Amazon Web Solutions (AWS), Google Cloud System (GCP), and Microsoft Azure Permit you to hire servers and services as you'll need them. You don’t must get components or guess foreseeable future ability. When website traffic improves, you could add more resources with just a few clicks or automatically utilizing automobile-scaling. When targeted traffic drops, it is possible to scale down to save cash.
These platforms also supply providers like managed databases, storage, load balancing, and safety resources. You are able to concentrate on developing your app in lieu of running infrastructure.
Containers are A different critical Device. A container packages your app and all the things it must operate—code, libraries, options—into a single unit. This can make it uncomplicated to move your app concerning environments, from the laptop to the cloud, without the need of surprises. Docker is the most well-liked Instrument for this.
When your application makes use of numerous containers, applications like Kubernetes help you take care of them. Kubernetes handles deployment, scaling, and recovery. If one section of the app crashes, it restarts it mechanically.
Containers also ensure it is easy to individual elements of your application into providers. You can update or scale areas independently, that is perfect for efficiency and trustworthiness.
In brief, applying cloud and container applications implies you can scale rapidly, deploy easily, and Recuperate quickly when troubles happen. If you need your application to develop devoid of limits, start off using these equipment early. They help you save time, decrease possibility, and help you remain focused on creating, not correcting.
Monitor Every little thing
When you don’t monitor your application, you gained’t know when points go wrong. Monitoring will help the thing is how your application is carrying out, place troubles early, and make improved decisions as your app grows. It’s a crucial Component of setting up scalable techniques.
Start out by monitoring basic metrics like CPU usage, memory, disk Area, and response time. These let you know how your servers and companies are executing. Applications like Prometheus, Grafana, Datadog, or New Relic can help you collect and visualize this information.
Don’t just check your servers—keep an eye on your application way too. Control how much time it will require for buyers to load internet pages, how frequently faults materialize, and exactly where they take place. Logging resources like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will help you see what’s taking place inside your code.
Setup alerts for essential issues. For instance, In case your response time goes higher than a Restrict or maybe a provider goes down, you must get notified quickly. This aids you resolve problems fast, often right before people even observe.
Checking can be beneficial whenever you make changes. For those who deploy a different attribute and see a spike in faults or slowdowns, you may roll it back again before it results in true injury.
As your application grows, website traffic and info increase. Without the need of monitoring, you’ll miss indications of difficulty right until it’s way too late. But with the proper applications set up, you remain on top of things.
In brief, checking aids you keep the app reliable and scalable. It’s not almost recognizing failures—it’s about comprehension your system and making certain it works properly, even stressed.
Ultimate Views
Scalability isn’t just for major organizations. Even compact apps need to have a strong foundation. By building very carefully, optimizing sensibly, and using the appropriate resources, you could Develop applications that grow easily devoid of breaking under pressure. Commence smaller, think massive, and Establish intelligent.